A React Native smart LED Control Adventure
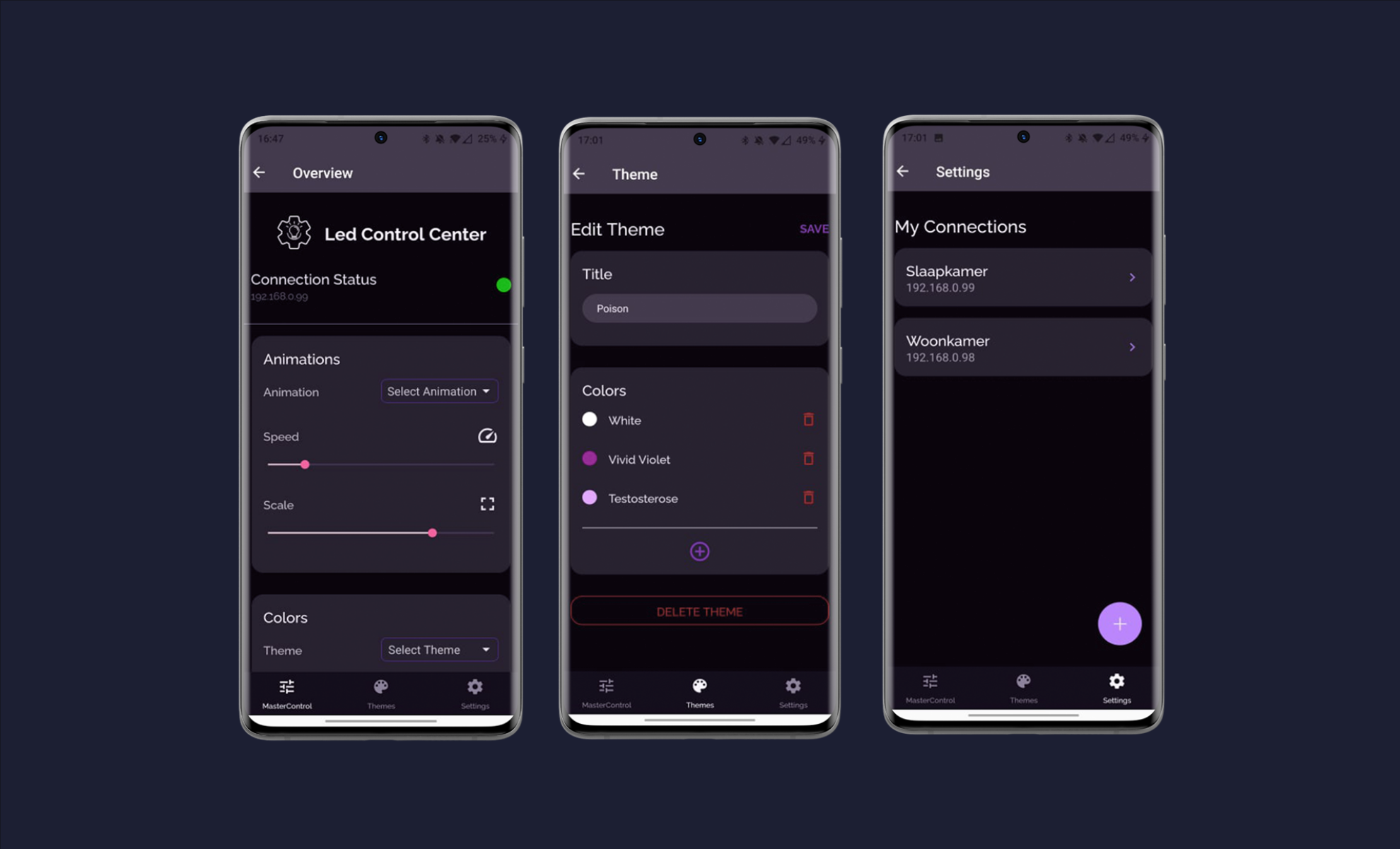
📝 Project description
Among the various projects I have done, one that stands out is a mobile application built using React Native and Expo. This application serves as a user-friendly interface to control a digital LED strip over Wi-Fi, with the LED strip connected to a Raspberry Pi.
The app has lots of practical features, allowing users to create custom themes and animations without much effort. Whether you're looking to set a specific ambiance or experiment with dynamic lighting, this application provides a seamless solution.
One of its notable functionalities is the ability to save and send custom themes directly to the Raspberry Pi over Wi-Fi. It is a great convenience of adjusting your LED strip's settings and designs with just a few taps on your mobile device.
🚨 LED controller
The primary objective of this project wasn't to develop a full-stack application. Instead, the focus was on explore React's mobile capabilities through React Native. Given the complexities of controlling a digital LED-strip, it was intentionally kept outside the project's scope.
To tackle the Raspberry Pi's role as a controller, I leveraged an open-source project available on Github (jackw01/led-control). This project aligned closely with my vision, offering a front-end UI for controlling a digital LED-strip. However, my goal was to craft a native application, distinguishing my approach from the existing project.
Upon reverse engineering, I uncovered that the project exposes a REST API for sending commands and themes to the LED-strip. This served as the foundation for my application, allowing seamless integration and control of the LED lights.
🔗 App features
The application has 3 main features that allow the user to control their LED-strip as conveniently as possible.
Connection management
The application retains a memory of connected devices or IP addresses, simplifying the process of managing multiple Raspberry Pis connected to LED strips on the same Wi-Fi network..
Master Controls
At the application's main screen lies the master controls, offering a centralized hub for managing various aspects. Here, you can easily control the animation type, animation speed, animation scale, select a theme, and adjust brightness, color temperature, and saturation.
Themes
Adding to its versatility, the app features a theme creation functionality. Users can craft custom themes by configuring their own color palettes, saving them for future use within the master controls.
The main screen, edit theme screen and connection management screen.
📈 Dataflow
To manage persistent data, the app relies on Expo SQLite, storing essential information such as connections (in one table) and themes (in two tables with a one-to-many relationship between palettes and colors).
export const initPalettes = async () => {
const db = getDb();
const tx = await transaction(db).catch(error => console.error(error));
if (tx) {
const res = await query(tx, {
sql: `CREATE TABLE IF NOT EXISTS palettes (
id integer NOT NULL PRIMARY KEY ,
name varchar(100)
);`,
args: []
})
}
}
export const initColors = async () => {
const db = getDb();
const tx = await transaction(db).catch(error => console.error(error));
if (tx) {
const res = await query(tx, {
sql: `CREATE TABLE IF NOT EXISTS color (
h double(10,20) NOT NULL ,
s double(10,20) NOT NULL ,
v double(10,20) NOT NULL ,
paletteId integer ,
colorId integer NOT NULL PRIMARY KEY autoincrement ,
FOREIGN KEY ( paletteId ) REFERENCES palette( id )
);`,
args: []
})
}
}
On the other hand, React Redux is used to handle state management like keeping track of the current connection, the current connection state (connection failed, succeeded) and other data coming from the database.
🧑💻 Source code
If you want to have a look at the source code of this project, you can visit Github repository of the project.